How to Create a Localization System in Unity
- Mert Karagöz
- Jul 9, 2022
- 2 min read
Updated: Jan 14, 2023
Localization is an important concept if you publish your game internationally. There are many ways to implement this feature, but from my perspective doing the job with a csv. file is more convenient than others.
In Unity, you can implement a localization system by using the built-in localization system or by using third-party localization tools. Here’s an example of how you can implement localization using the built-in localization system in Unity:
1.Create a new folder in your project called “Localization” and create a new file called “Localization.csv” in this folder.
Here is an example of a .csv file that could be used for localization in a Unity game:
Key ,English ,French
Title, Welcome to our game!,Bienvenue dans notre jeu!
StartButton, Start Game ,Démarrer le jeu
OptionsButton, Options ,Options
QuitButton, Quit ,Quitter
This file contains four columns: “Key,” “English,” and “French,” Each row in the file represents a different localization string. The first column, “Key,” contains a unique identifier for each string. The remaining columns contain the localized versions of the string for each language. In this example, the localized strings are provided for English, French, and German.
You can import this file in Unity and use it to dynamically change the text in your game depending on the player’s language preference.
2.In the “Localization.csv” file, create a table of all the text that needs to be localized in your game. The first row should contain the column headers (e.g. “Key”, “English”, “French”, etc.) and each subsequent row should contain a unique key for the text and the corresponding text in each language.
3.In Unity, go to the “Edit” menu and select “Project Settings” and then “Localization”. In the Localization settings, select “Import” and select the “Localization.csv” file. Unity will automatically create a localization database and import the text from the csv file.
4.Create a new script and attach it to a GameObject in your scene. In this script, you can use the Localization.Get function to retrieve localized text by key.
using UnityEngine;
using UnityEngine.Localization;
public class LocalizationManager : MonoBehaviour
{
public string key;
private void Start()
{
string localizedText = Localization.Get(key);
Debug.Log(localizedText);
}
}
In this example, the script takes a string parameter called “key” and uses it to retrieve the corresponding localized text using the Localization.Get function. You can then use this text to display in a Text component or any other UI element.
5.In order to change the language of your game, you can use the Localization.CurrentLanguage property to set the current language.
Localization.CurrentLanguage = SystemLanguage.Turkish;
This is a basic example of how to use the built-in localization system in Unity, but there are many third-party localization tools available that can provide more advanced features such as real-time editing, automatic translation, and more.
See you next time,
You can access the original article from the link below:
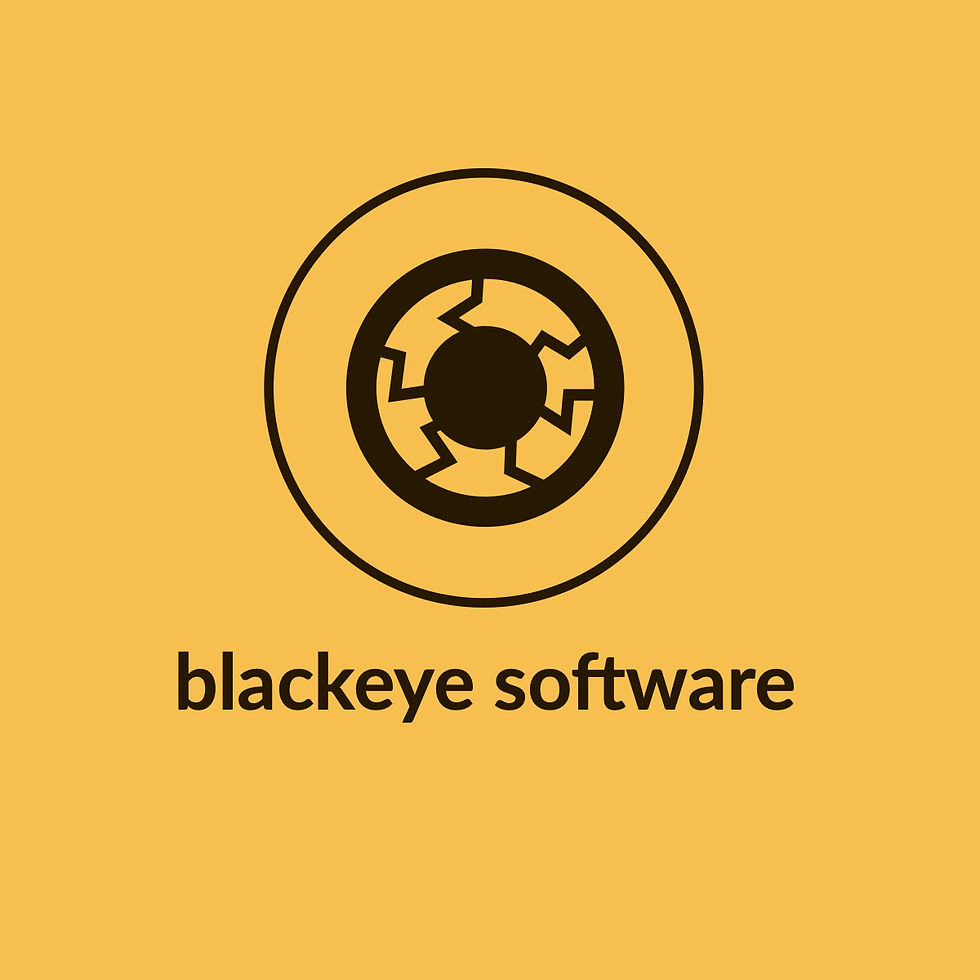
Comentários